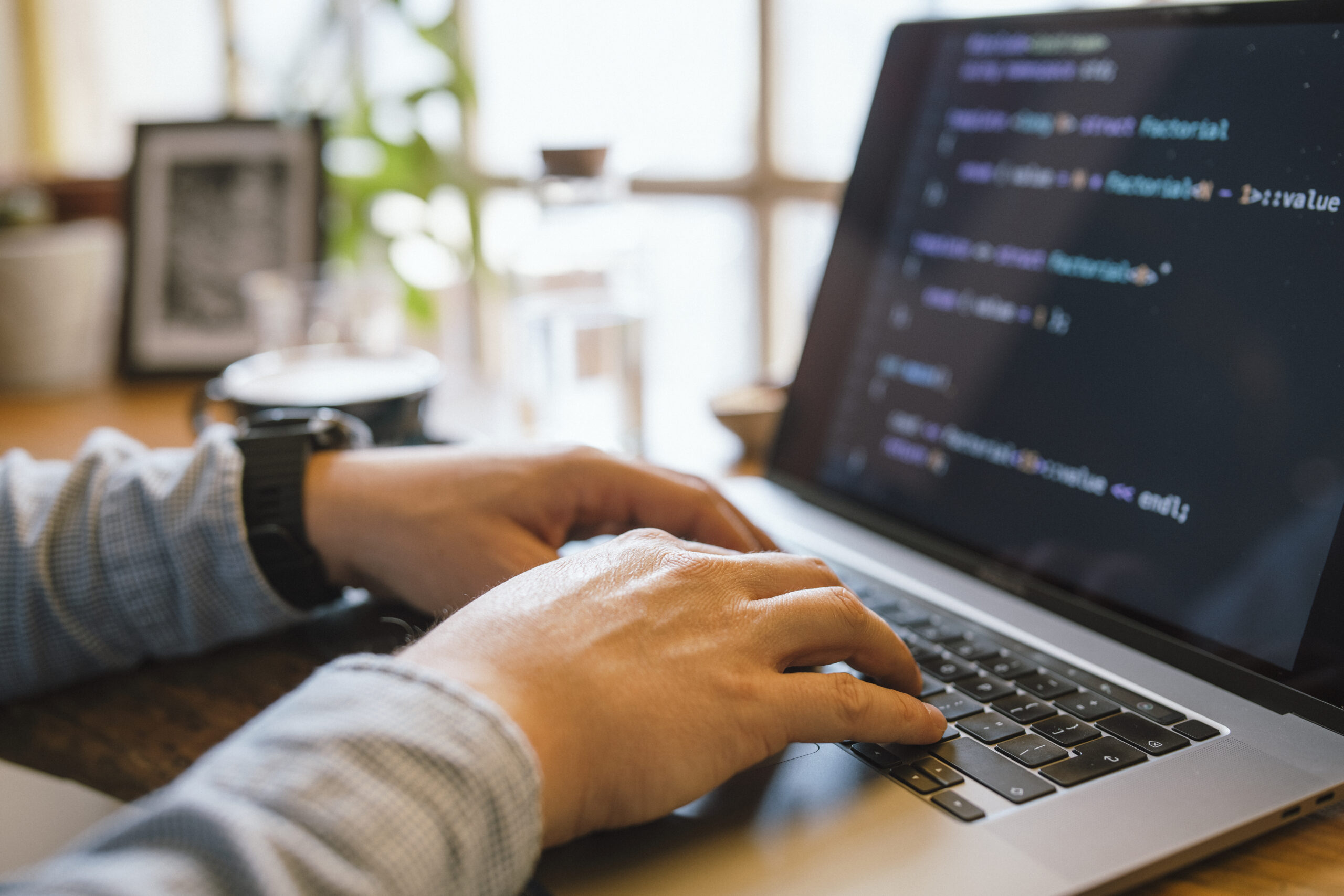
Debugging is Just about the most essential — but typically missed — abilities inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productiveness. Listed below are numerous methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
Among the quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While producing code is just one Section of improvement, knowing ways to communicate with it properly in the course of execution is equally significant. Modern-day growth environments come equipped with impressive debugging abilities — but numerous builders only scratch the surface area of what these applications can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools permit you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code within the fly. When employed correctly, they Enable you to notice specifically how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, check community requests, see authentic-time overall performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can turn aggravating UI challenges into manageable duties.
For backend or process-level builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than managing procedures and memory administration. Understanding these resources could have a steeper learning curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Further than your IDE or debugger, come to be at ease with Edition Management units like Git to understand code background, find the exact minute bugs were released, and isolate problematic modifications.
In the end, mastering your equipment signifies going beyond default settings and shortcuts — it’s about building an intimate understanding of your growth natural environment to ensure that when problems come up, you’re not misplaced at midnight. The better you realize your instruments, the greater time it is possible to commit fixing the actual difficulty as opposed to fumbling by the method.
Reproduce the challenge
The most vital — and often ignored — steps in efficient debugging is reproducing the issue. Before leaping into your code or building guesses, developers require to make a constant environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug results in being a video game of likelihood, frequently bringing about wasted time and fragile code modifications.
The initial step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps led to The difficulty? Which natural environment was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it becomes to isolate the precise circumstances less than which the bug happens.
Once you’ve gathered enough information, try and recreate the issue in your neighborhood atmosphere. This might mean inputting precisely the same info, simulating identical consumer interactions, or mimicking procedure states. If the issue seems intermittently, take into consideration composing automated exams that replicate the sting instances or point out transitions involved. These exams not simply assist expose the problem but in addition reduce regressions in the future.
Often, The difficulty might be setting-particular — it would materialize only on certain working programs, browsers, or less than specific configurations. Making use of tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a move — it’s a mindset. It calls for endurance, observation, in addition to a methodical solution. But once you can constantly recreate the bug, you are now midway to correcting it. With a reproducible scenario, You should use your debugging resources a lot more proficiently, exam opportunity fixes properly, and connect extra Evidently with all your workforce or users. It turns an abstract criticism right into a concrete problem — and that’s in which developers thrive.
Go through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then seeing them as frustrating interruptions, builders really should understand to treat mistake messages as immediate communications from your process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you know the way to interpret them.
Start off by reading through the message diligently and in complete. Lots of developers, particularly when below time tension, glance at the first line and straight away start off creating assumptions. But further from the error stack or logs may perhaps lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and realize them first.
Split the mistake down into areas. Can it be a syntax error, a runtime exception, or simply a logic error? Does it place to a particular file and line range? What module or perform activated it? These concerns can tutorial your investigation and stage you towards the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Finding out to acknowledge these can significantly hasten your debugging system.
Some mistakes are obscure or generic, As well as in those conditions, it’s essential to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
Ultimately, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When used properly, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s occurring beneath the hood while not having to pause execution or phase in the code line by line.
A very good logging system starts off with recognizing what to log and at what amount. Prevalent logging degrees incorporate DEBUG, Data, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info throughout development, Facts for typical gatherings (like thriving start off-ups), Alert for prospective problems that don’t break the applying, ERROR for actual problems, and FATAL when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your method. Focus on critical activities, state improvements, input/output values, and important determination points within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without halting the program. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, smart logging is about equilibrium and clarity. Having a very well-thought-out logging method, it is possible to lessen the time it will take to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a complex endeavor—it is a type of investigation. To properly establish and fix bugs, developers need to technique the procedure like a detective solving a thriller. This frame of mind can help stop working complex problems into manageable elements and comply with clues logically to uncover the root cause.
Begin by gathering evidence. Look at the signs of the trouble: error messages, incorrect output, or functionality difficulties. Much like a detective surveys a crime scene, collect as much relevant info as you are able to with out jumping to conclusions. Use logs, test cases, and consumer reviews to piece with each other a clear picture of what’s going on.
Future, sort hypotheses. Check with by yourself: What may be producing this habits? Have any alterations just lately been created for the codebase? Has this problem occurred right before underneath related situations? The aim would be to narrow down alternatives and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled surroundings. In the event you suspect a selected operate or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome direct you nearer to the truth.
Pay near interest to compact specifics. Bugs often cover within the the very least predicted locations—just like a missing semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The problem with out fully comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on That which you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can conserve time for foreseeable future issues and aid Many others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden problems in intricate units.
Create Exams
Producing tests is one of the best strategies to help your debugging skills and All round growth effectiveness. Assessments don't just help catch bugs early and also function a security Web that offers you assurance when making modifications for your codebase. A effectively-examined application is simpler to debug mainly because it helps you to pinpoint exactly the place and when a challenge happens.
Begin with unit exams, which give attention to particular person capabilities or modules. These smaller, isolated tests can rapidly reveal whether a specific bit of logic is Doing the job as envisioned. Every time a examination fails, you quickly know in which to search, appreciably minimizing time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Future, combine integration exams and end-to-close assessments into your workflow. These aid make sure that various portions of your software perform together efficiently. They’re significantly valuable for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which Element of the pipeline failed and under what conditions.
Composing tests also forces you to definitely think critically regarding your code. To test a attribute correctly, you would like to comprehend its inputs, envisioned outputs, and edge instances. This volume of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you'll be able to deal with fixing the bug and look at your exam pass when The problem is solved. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing exams turns debugging from the disheartening guessing game into a structured and predictable approach—serving to you capture more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lower irritation, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain becomes less efficient at problem-resolving. A brief stroll, a coffee crack, or simply switching to a different task for ten–15 minutes can refresh your target. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing Gustavo Woltmann AI their subconscious perform within the history.
Breaks also help reduce burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is not only unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You may instantly observe a missing semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute crack. Use that time to maneuver about, extend, or do something unrelated to code. It could feel counterintuitive, Primarily below limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is usually a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is much more than simply A short lived setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural concern, each can train you a thing valuable in the event you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be a great behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or prevalent problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from the bug with the peers may be Primarily highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts staff effectiveness and cultivates a much better learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce excellent code, but individuals that continually master from their faults.
In the end, Every single bug you fix adds a completely new layer in your talent established. So up coming time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and persistence — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a possibility to be better at Everything you do.